Reset Webflow Form
How to use:
Unlock more with PRO
Want to learn how to use this Crumb? Unlock detailed documentation, video tutorials, comments & support!
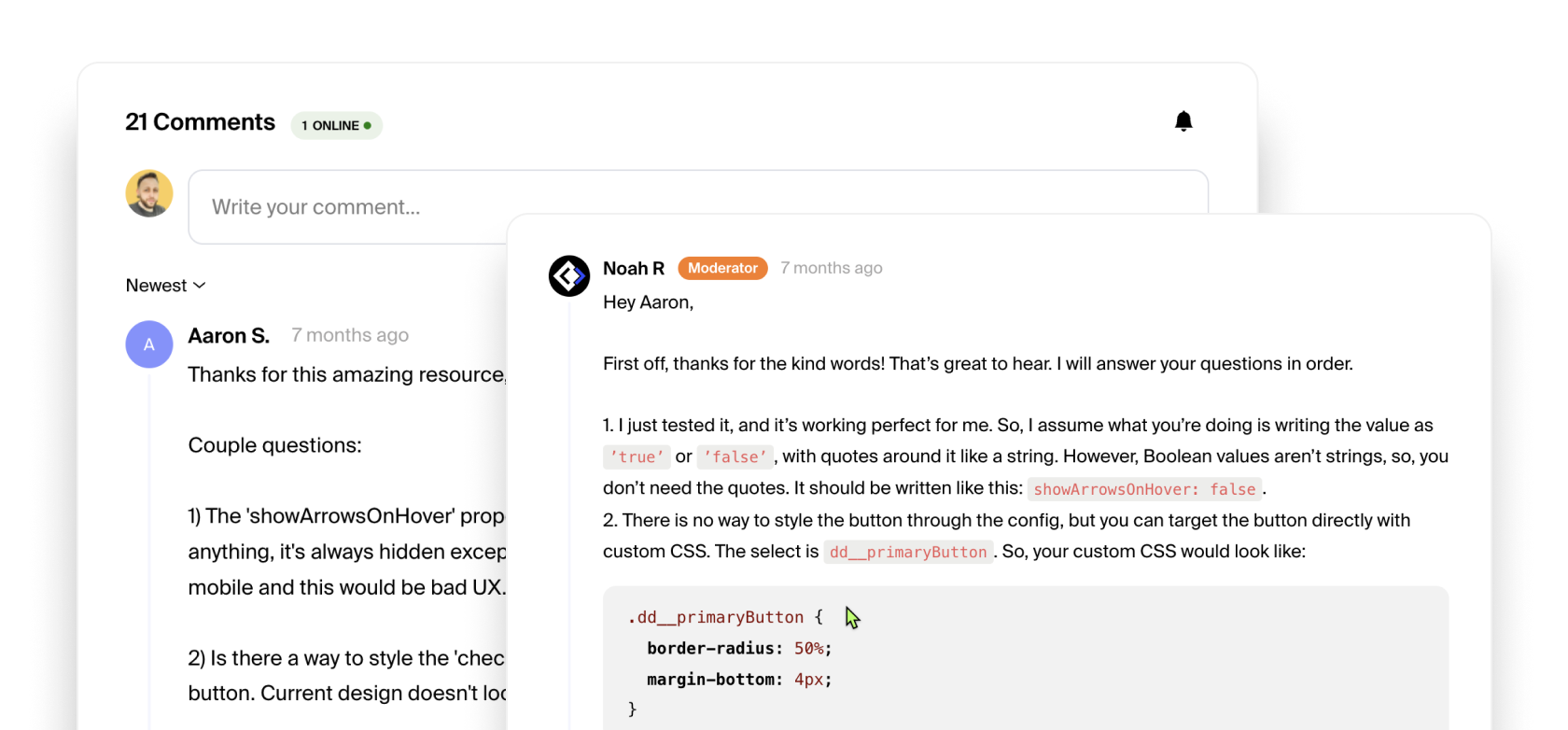
Unlock more with PRO
Want to learn how to use this Crumb? Unlock detailed documentation, video tutorials, comments & support!
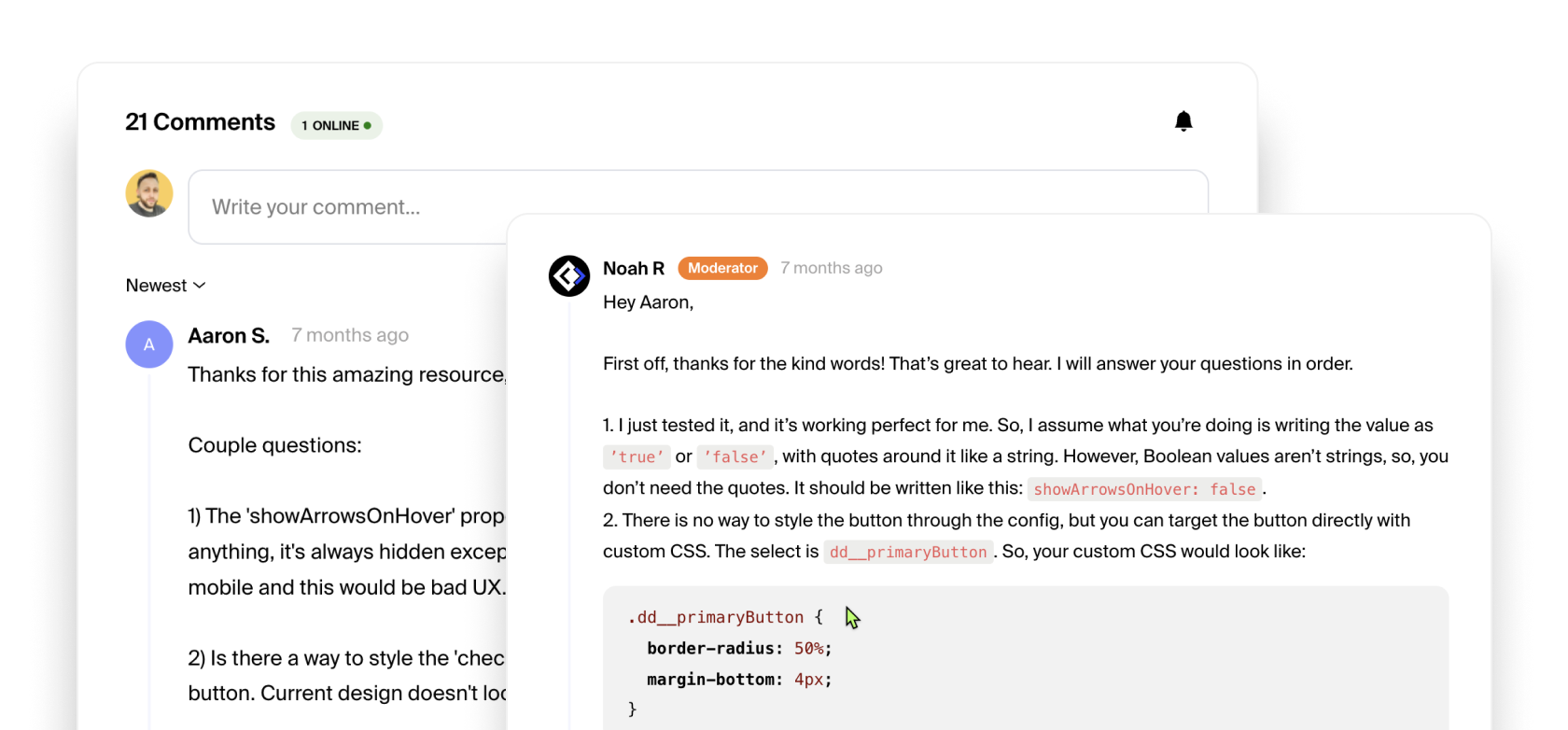
Once you've pasted the code into your </ body> custom code section of the page settings, all you have to do is change the selector name in the code to match your forms selector name. This can be an id (#) or a class name (.) as show below.

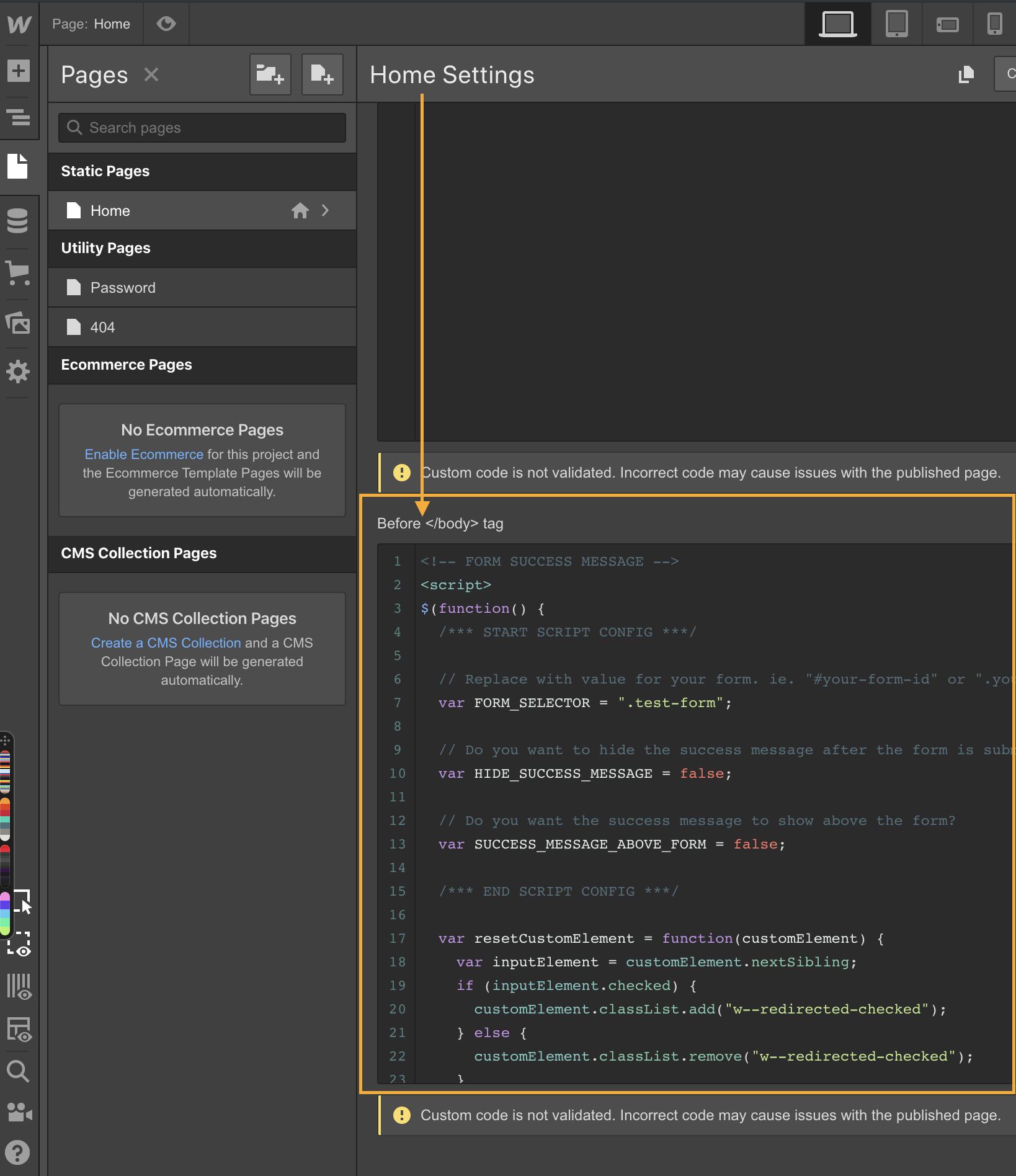
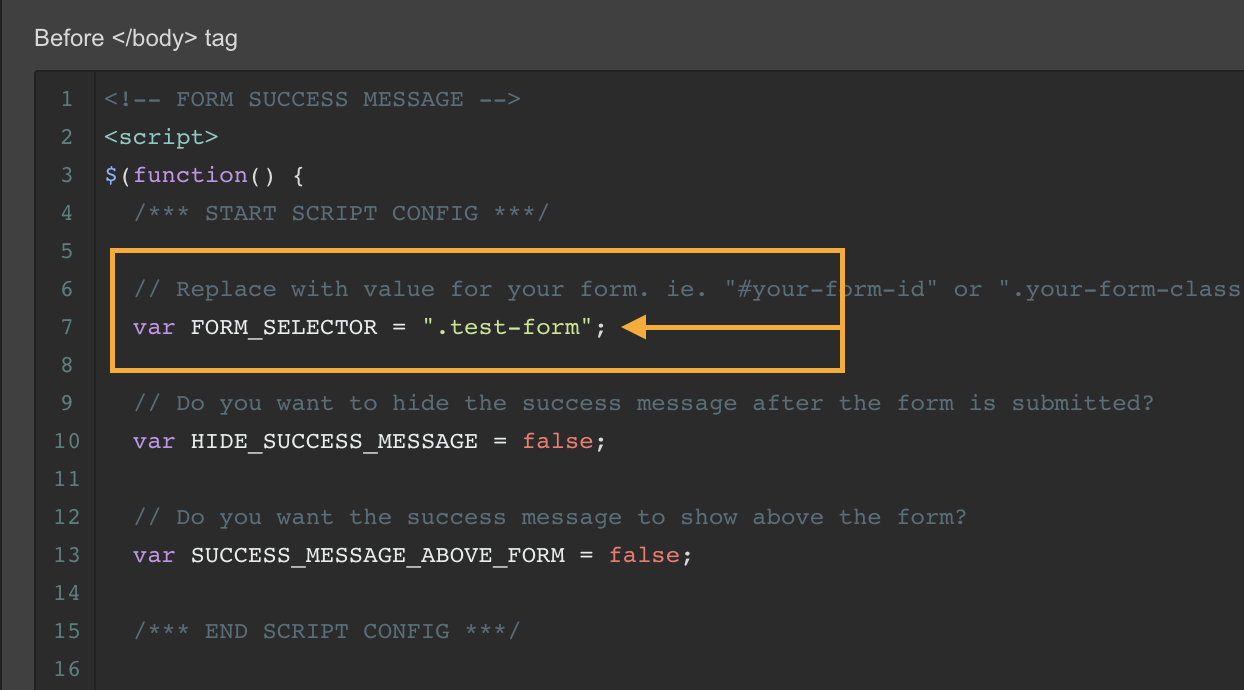
We have another cool option to either show the success message on top or below the form. The value uses a boolean (true or false). In this case we selected false to show the success message below the form after submit. The last option we're given is to show or hide the success message altogether (also using a boolean value, true or false).
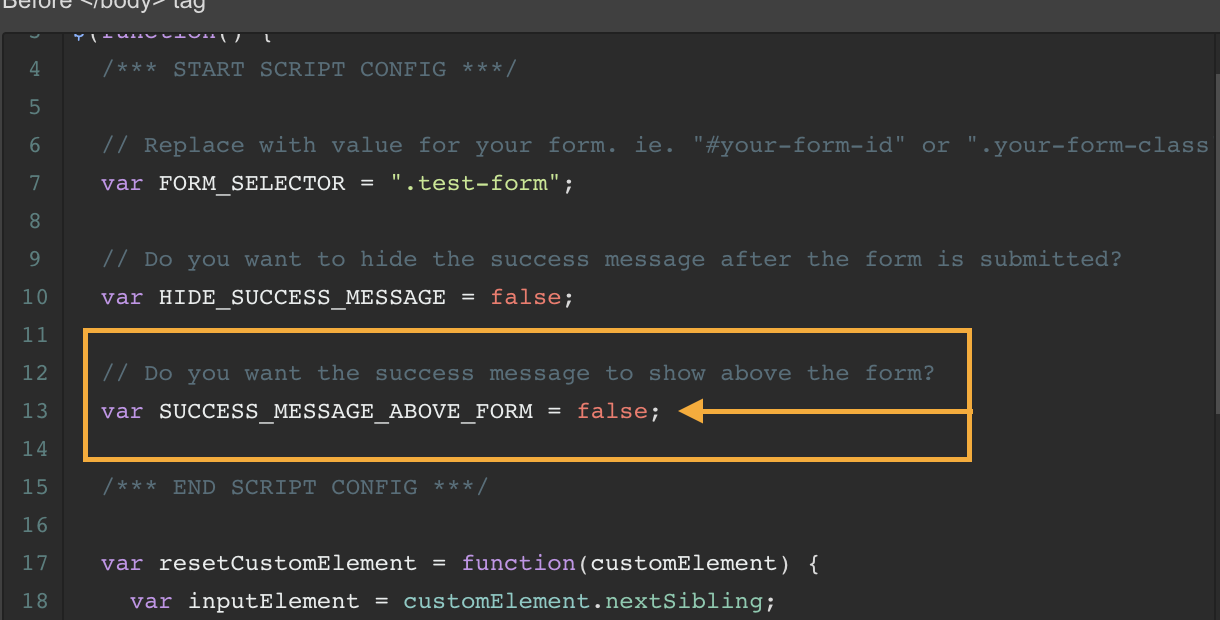
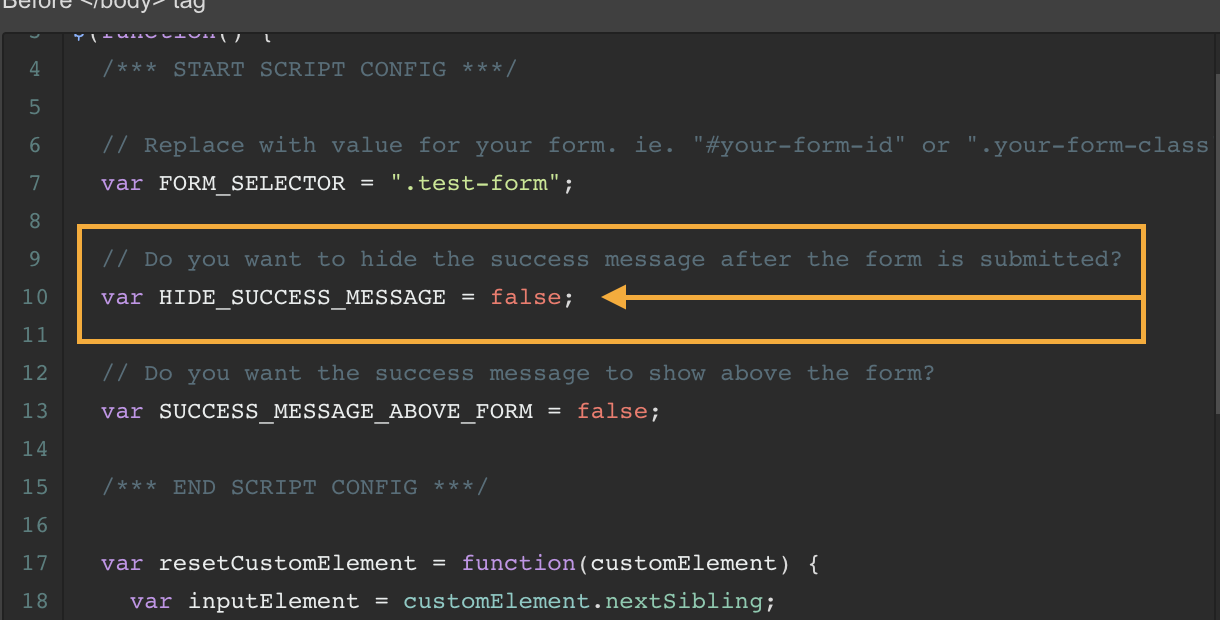
Lastly, if you noticed in the demo we added a close (X) button for the success message. This is done simply by adding a link-block or div (whatever you want to use for a button really) and added a very simple Webflow interaction to hide the message with a "Click or Tap" trigger. That's all. 🤙